Creating Layout at runtime
UI Component can be created programmatically. UI component class setter methods help to configure the component. This style is not recommended unless it’s really required. In this style, business logic gets mixed with the component UI code. It doesn’t look neat and sometimes it’s hard to manage.
Relative Layout
package androiindians.dynamic;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.widget.RelativeLayout;
public class MainActivity extends AppCompatActivity {
RelativeLayout rl;
RelativeLayout.LayoutParams param1, param2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
rl = new RelativeLayout(MainActivity.this);
param1 = new RelativeLayout.LayoutParams((int) RelativeLayout.LayoutParams.WRAP_CONTENT,
(int) RelativeLayout.LayoutParams.WRAP_CONTENT);
param2 = new RelativeLayout.LayoutParams((int) RelativeLayout.LayoutParams.WRAP_CONTENT,
(int) RelativeLayout.LayoutParams.WRAP_CONTENT);
param1.leftMargin = 100;
param1.topMargin = 100;
param2.setMargins(170, 200, 0, 0);
setContentView(rl);
}
}
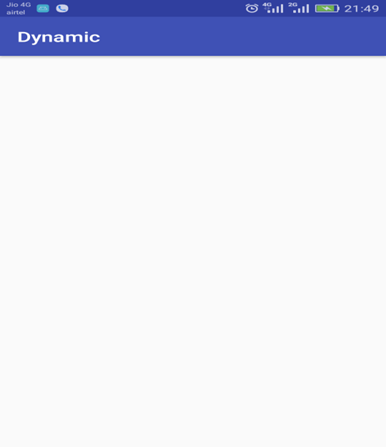