Activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent"
android:orientation="vertical" >
<Button
android:id="@+id/buttonClick"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click To Show Custom Dialog" />
</LinearLayout>
custom.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="fill_parent"
android:layout_height="fill_parent" >
<ImageView
android:id="@+id/imageDialog"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginRight="6dp" />
<TextView
android:id="@+id/textDialog"
android:layout_width="fill_parent"
android:layout_height="wrap_content"
android:textColor="#FFF"
android:layout_toRightOf="@+id/imageDialog"/>
<Button
android:id="@+id/declineButton"
android:layout_width="100px"
android:layout_height="wrap_content"
android:text=" Submit "
android:layout_marginTop="5dp"
android:layout_marginRight="5dp"
android:layout_below="@+id/textDialog"
android:layout_toRightOf="@+id/imageDialog"
/>
</RelativeLayout>
MainActivity.java
package androiindians.customlist;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import android.app.Activity;
import android.app.Dialog;
import android.os.Bundle;
import android.view.View;
import android.view.View.OnClickListener;
public class MainActivity extends Activity {
private Button buttonClick;
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
buttonClick = (Button) findViewById(R.id.buttonClick);
// add listener to button
buttonClick.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View arg0) {
// Create custom dialog object
final Dialog dialog = new Dialog(CustomDialog.this);
// Include dialog.xml file
dialog.setContentView(R.layout.dialog);
// Set dialog title
dialog.setTitle("Custom Dialog");
// set values for custom dialog components - text, image and button
TextView text = (TextView) dialog.findViewById(R.id.textDialog);
text.setText("Custom dialog Android example.");
ImageView image = (ImageView) dialog.findViewById(R.id.imageDialog);
image.setImageResource(R.drawable.image0);
dialog.show();
Button declineButton = (Button) dialog.findViewById(R.id.declineButton);
// if decline button is clicked, close the custom dialog
declineButton.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
// Close dialog
dialog.dismiss();
}
});
}
});
}
}
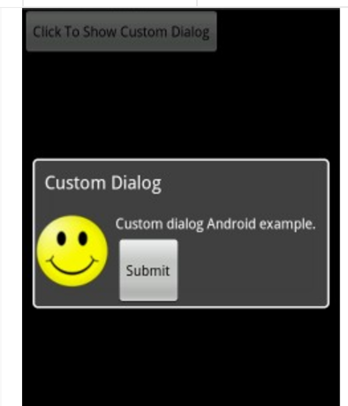