In Android, RelativeLayout let you position your component base on the nearby (relative or sibling) component’s position. It’s the most flexible layout, that allow you to position your component to display in anywhere you want (if you know how to “relative” it).
In RelativeLayout, you can use “above, below, left and right” to arrange the component position, for example, display a “button1” below “button2”, or display “button3” on right of the “button1”.
Note: The RelativeLayout is very flexible, but hard to master it. Suggest you use Studio IDE to drag the component, then view study the Studio generated XML layout code to understand how to code “relative” components. Example code & properties of Relative Layout
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginBottom="31dp"
android:text="Button" />
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentBottom="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:layout_marginBottom="31dp"
android:text="Button" />
<Button
android:id="@+id/button3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentTop="true"
android:layout_marginLeft="37dp"
android:layout_marginStart="37dp"
android:layout_toEndOf="@+id/button"
android:layout_toRightOf="@+id/button"
android:text="Button" />
<Button
android:id="@+id/button4"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button3"
android:layout_marginLeft="157dp"
android:layout_marginStart="157dp"
android:layout_marginTop="77dp"
android:layout_toEndOf="@+id/button3"
android:layout_toRightOf="@+id/button3"
android:text="Button" />
<Button
android:id="@+id/button5"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_below="@+id/button3"
android:layout_centerHorizontal="true"
android:layout_marginTop="14dp"
android:text="Button" />
<Button
android:id="@+id/button6"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignEnd="@+id/button5"
android:layout_alignRight="@+id/button5"
android:layout_alignTop="@+id/button5"
android:text="Button" />
<Button
android:id="@+id/button7"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="32dp"
android:text="Button"
android:layout_marginRight="57dp"
android:layout_marginEnd="57dp"
android:layout_alignTop="@+id/button4"
android:layout_alignParentRight="true"
android:layout_alignParentEnd="true" />
<Button
android:id="@+id/button8"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignParentEnd="true"
android:layout_alignParentRight="true"
android:layout_below="@+id/button4"
android:layout_marginTop="38dp"
android:text="Button" />
</RelativeLayout>
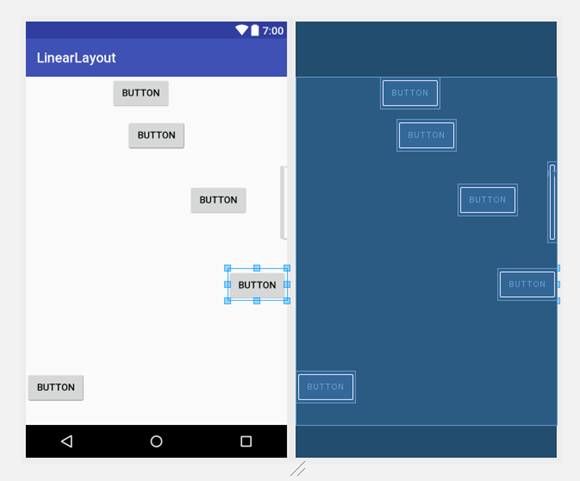
Properties of Relative Layout
android:id: This is the ID which uniquely identifies the layout
android:gravity: This specifies how an object should position its content, on both the X and Y axes. Possible values are top, bottom, left, right, center, center_vertical, center_horizontal etc
android:ignoreGravity: This indicates what view should not be affected by gravity.
android:layout_above: The bottom edge of this view above the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”
android:layout_alignBottom: Makes the bottom edge of this view match the bottom edge of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_alignLeft: Makes the left edge of this view match the left edge of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_alignParentBottom: If true, makes the bottom edge of this view match the bottom edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignParentEnd : If true, makes the end edge of this view match the end edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignParentLeft : If true, makes the left edge of this view match the left edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignParentRight: If true, makes the right edge of this view match the right edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignParentStart: If true, makes the start edge of this view match the start edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignParentTop : If true, makes the top edge of this view match the top edge of the parent. Must be a boolean value, either “true” or “false”.
android:layout_alignRight: Makes the right edge of this view match the right edge of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_alignStart: Makes the start edge of this view match the start edge of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_alignTop: Makes the top edge of this view match the top edge of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_below: The top edge of this view below the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_centerHorizontal: If true, centers this child horizontally within its parent. Must be a boolean value, either “true” or “false”.
android:layout_centerInParent: If true, centers this child horizontally and vertically within its parent. Must be a boolean value, either “true” or “false”.
android:layout_centerVertical: If true, centers this child vertically within its parent. Must be a boolean value, either “true” or “false”.
android:layout_toEndOf: Positions the start edge of this view to the end of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_toLeftOf: Positions the right edge of this view to the left of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_toRightOf: Positions the left edge of this view to the right of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.
android:layout_toStartOf: Positions the end edge of this view to the start of the given anchor view ID and must be a reference to another resource, in the form “@[+][package:]type:name”.