Registration API
Url: http://androindian.com/test/Register.php
Request: Form Data
Keys are username, email, password
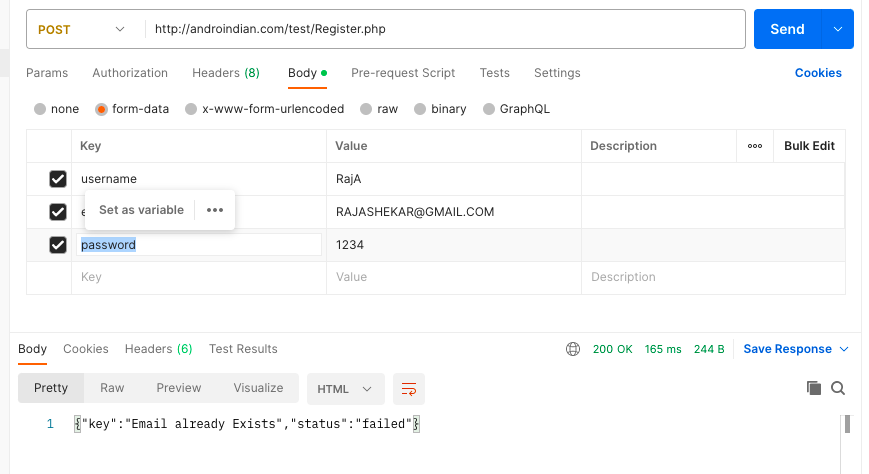
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<layout
xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
tools:context=".MainActivity">
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/username"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPersonName"
android:hint="UserName"/>
<EditText
android:id="@+id/mail"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textEmailAddress"
android:hint="mail"/>
<EditText
android:id="@+id/password"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:ems="10"
android:inputType="textPassword"
android:hint="Password"/>
<Button
android:id="@+id/reg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Reg" />
</LinearLayout>
</layout>
MainActivity.java
package com.androindian.retro;
import androidx.appcompat.app.AppCompatActivity;
import androidx.databinding.DataBindingUtil;
import android.os.Bundle;
import android.util.Log;
import android.view.View;
import android.widget.Toast;
import com.androindian.retro.databinding.ActivityMainBinding;
import com.google.gson.JsonObject;
import java.util.Objects;
import okhttp3.MultipartBody;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
import retrofit2.http.Multipart;
public class MainActivity extends AppCompatActivity {
ActivityMainBinding binding;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
binding= DataBindingUtil.setContentView(
MainActivity.this,R.layout.activity_main);
binding.reg.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
MultipartBody.Part name = MultipartBody.Part.createFormData
("username", Objects.requireNonNull(binding.username.getText().toString().trim()));
MultipartBody.Part mail = MultipartBody.Part.createFormData(
"email",binding.mail.getText().toString().trim()
);
MultipartBody.Part pass = MultipartBody.Part.createFormData
("password",binding.password.getText().toString().trim());
Retrofit retrofit=new Retrofit.Builder()
.baseUrl("https://androindian.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
RetroInterface retroInterface=retrofit.create(RetroInterface.class);
Call<RegResponse> regResponseCall=retroInterface.createUser(name,mail,pass);
regResponseCall.enqueue(new Callback<RegResponse>() {
@Override
public void onResponse(Call<RegResponse> call,
Response<RegResponse> response) {
Log.e("response",String.valueOf(response.body()));
String res=response.body().getKey();
if(res!=null){
Toast.makeText(MainActivity.this, "Reg Sucess", Toast.LENGTH_SHORT).show();
}else {
Toast.makeText(MainActivity.this, "Reg failed", Toast.LENGTH_SHORT).show();
}
}
@Override
public void onFailure(Call<RegResponse> call,
Throwable t) {
Toast.makeText(MainActivity.this, ""+t.toString(), Toast.LENGTH_SHORT).show();
}
});
}
});
}
}
RetroInterface.java
package com.androindian.retro;
import com.google.gson.JsonObject;
import okhttp3.MultipartBody;
import retrofit2.Call;
import retrofit2.http.Body;
import retrofit2.http.Headers;
import retrofit2.http.Multipart;
import retrofit2.http.POST;
import retrofit2.http.Part;
public interface RetroInterface {
@Multipart
@Headers("Content-Type:application/json")
@POST("test/Register.php")
Call<RegResponse> createUser(@Part MultipartBody.Part name, @Part MultipartBody.Part email, @Part MultipartBody.Part password);
}
RegResponse.Java
package com.androindian.retro;
import com.google.gson.annotations.Expose;
import com.google.gson.annotations.SerializedName;
public class RegResponse {
@SerializedName("key")
@Expose
private String key;
@SerializedName("status")
@Expose
private String status;
public String getKey() {
return key;
}
public void setKey(String key) {
this.key = key;
}
public String getStatus() {
return status;
}
public void setStatus(String status) {
this.status = status;
}
}
androidManifest.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools">
<uses-permission android:name="android.permission.INTERNET"></uses-permission>
<application
android:allowBackup="true"
android:dataExtractionRules="@xml/data_extraction_rules"
android:fullBackupContent="@xml/backup_rules"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/Theme.RETRO"
tools:targetApi="31">
<activity
android:name=".MainActivity"
android:exported="true">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
Add below lines in build gradle
dataBinding{
enabled=true
}
dependencies {
implementation 'com.squareup.retrofit2:converter-gson:2.4.0'
implementation 'com.squareup.retrofit2:retrofit:2.4.0'
implementation 'com.google.code.gson:gson:2.8.9'
}