String is a predefined class, which can be used for storing a group of characters.
To store a group of characters we need to create an object of String class. The String class object can be created in two ways.
1. The String class object can be created by using new operator
String str = new String(“hello”);
2. The String class object can be created by specifying a group of characters enclosed directly in a pair of double quotes(” “).
String str = “hello”;
The String objects created by both the mechanisms are called as immutable object, which means once the String object is created we cannot modify the content of the object.
Difference between the two mechanisms of creating String objects:
String Object Created by using new Operator
1) Location: The String objects created by using new operator are stored in heap memory
2) Allocation: if the String objects are stored in heap memory then, it will never verify, whether the heap memory contains objects with same content or not, it will always create new object and store the content
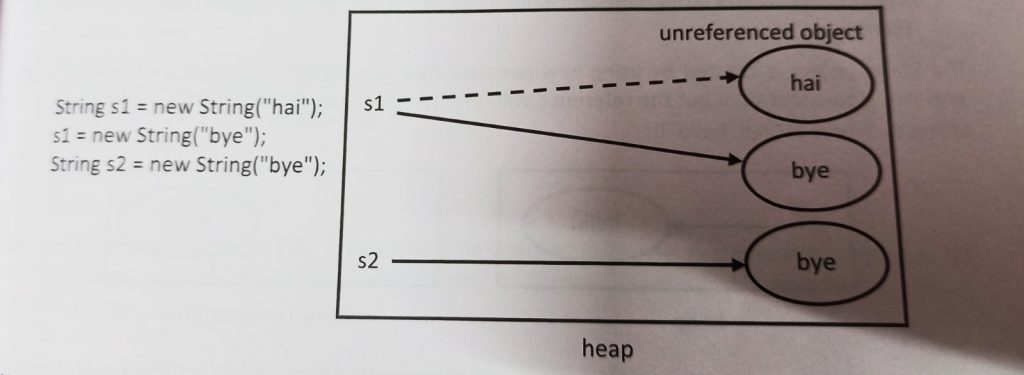
Unreferenced Object: If an object does not have any live reference ie. if no reference variable is referring to the object then, the object should be called as unreferenced object
3) Deallocation: If the heap memory contains unreferenced objects then, they will be deallocated by garbage collector
String object created by specifying a group of characters enclosed directly in a pair of double quotes:
1) Location: if a String object is created by enclosing a group of characters in a pair of double quotes (“”) then, they are stored in string constant pool.
2) Allocation: if the String objects are stored in string constant pool then, it will always verify whether the string constant pool contain objects with same content or not if available then. refer to existing object. If not available then create a new object
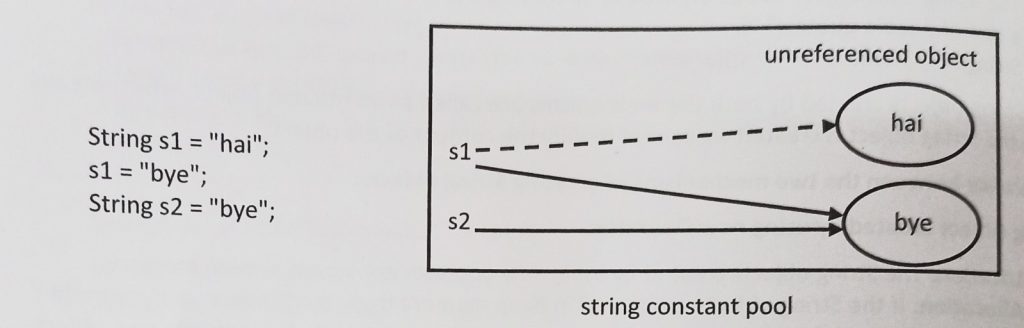
3) Deallocation: if the string constant pool contains unreferenced object then, they will be deallocated by the string constant pool itself, when the string constant pool is completely filled
If a String object is created by using new operator then the content will be stored in both heap and string constant pool but the reference will refer to the object available in heap memory. String s1 = new Stringi”hello”);
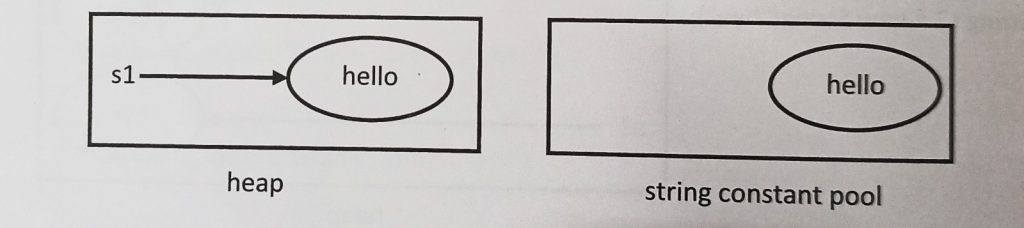
If a String object is created by specifying a group of characters enclosed directly in a pair of double quotes then the content will be stored in only in string constant pool String s1 = “hello”;
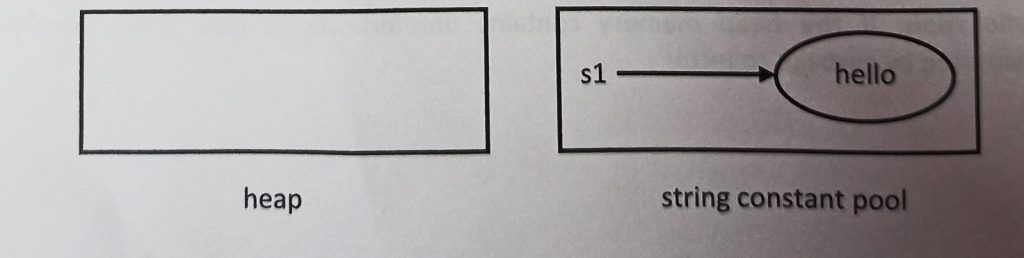
Method Of String Class:
- int length(): This method will return the count of the number of characters available in a String.
class String Demo {
public static void main(String[] args) {
String str = new String("java program");
System out printin (str length());
}
}
- char charAt(int index): This method will return a character that is available at the specified index position
The index position starts from 0. If the specified index is not within the range then, it will generate a runtime error called StringindexOutOfBoundsException
String str = new String! "java program");
System.out printin(str.charAt(5));
System.out.printin(str charAt(15));
3) String concat(String): This method can be used to append the contents of one string to
another string
String s1 = new String("java");
String s2 = new String("program");
s1= s1 concat (s2);
System.out printin(s1);
System out println(s2);
4) int compareTo(String): This method can be used to compare the unicode values of the characters available in a String, by considering their case This method is designed for sorting the strings
S1>S2 +VE
S1<S2 -VE
S1==S2 0
5) int compareTolgnoreCase(String): This method can be used to compare the unicode values of the characters available in a String by ignoring their case This method is designed for sorting the strings
Example:
String s1 = new String(“abc”)’;
String s2 = new String(“bcd”);
System.out.println(s1.compareTo(s2));
System.out.println(s1.compareTolgnoreCase(s2));
6) boolean equals(Object): This method can be used to compare the contents of the string objects by considering their case.
7) boolean equalsIgnoreCase(String): This method can be used to compare the content of the string objects by ignoring their case.
Example:
String s1 = new String(“abc”);
String s2 = new String(“ABC”);
System.out.println(s1.equals(s2));
System.out.println(s1.equalsIgnoreCase(s2));
8) boolean startsWith(String): This method can be used to check whether a string begins with a specified group of characters or not
9) boolean endsWith(String): This method can be used to check whether a string ends with a specified group of characters or not.
Note: The startsWith() and endsWith() will consider the case
Example:
String str = new String(“java program”);
System.out.println(str.startsWith(“ja”));
System.out.println(str.startsWith(“jab”));
System.out.println(str.startsWith(“JAVA”));
System.out.println(str.endsWith(“ram”));
System.out.println(str.endsWith(“Gram”));
10) int indexOf(char): This method will return the index of the first occurrence of the specified
character
11) int lastIndexOf: This method will return the index of the last occurrence of the specified character
Note: The indexOf() and lastindexOf() will return -1, if the specified character is not available.
Example:
String str = new String(“java program”);
System.out.println(str.indexOf(‘a’));
System.out.println(str.indexOf(‘p’));
System.out.println(str.indexOf(‘q’));
System.out.println(str.lastIndexOf(‘a’));
System.out.println(str.lastIndexOf(‘p’));
System.out.println(str.lastIndexOf(‘z’));
12) String replace(char old, char new): This method can be used to replace all the occurrences of the specified character with a new character
Example:
String str = new String(“java jug jar jungle”);
System.out.println(str.replace(‘j’, ‘b’));
13) String substring(int index): This method will return a group of characters beginning from the
specified index position up to the end of the string
14) String substring(int index, int offset): This method will return a group of characters beginning from the specified index position up to the specified offset
Note: The offset represents the position of a character in a string and it begins with 1
Example:
String str = new String(“java program”);
System.out.println(str.substring(3));
System.out.println(str.substring (2,9));
15) String toLowerCase(): This method will convert the contents of a string to completely lower case.
16) String toUpperCase(): This method will convert the contents of a string to completely upper case
Example:
String str = new String(“java program”);
System.out.println(str);
System.out.println(str.toLowerCase());
System.out.println(str.toUpperCase());
17) String trim(): This method can be used to renove the leading and trialing spaces available in a string
Example:
String str = new String(“java program”);
System.out.printin(str+”bye”);
System.out.println(str.trim()+”bye”);
18) String intern(): This method will refer to an object available in string constant pool which was created at the time of object creation in heap memory.
Example:
String s1 = new String(“hello”);
String s2 = s1.intern();
System.out.println(s1);
System.out.printin(s2);
Chaining Of Methods:
class Sample {
public static void main(String[] args) {
String str = new String("core");
System.out.println(str.concat("java").substring (4).concat("program")
.substring(4,8).concat("test").replace('z', 'n').toUpperCase().charAt(2));
}
}
Rule: If both the operands are of numeric type then + operator will perform addition and if atleast one operand is of string type then + operator will perform concatenation.
class Sample {
public static void main(String[] args) {
String str = new String("hello");
System.out.println(str+1+2+3);
System.out.println(1+str+2+3);
System.out.println 1+2+str+3);
System.out.println 1+2+3+str);
System.out.println( (1+2)+str+3);
System.out.println (1+str+(2+3))
}
}
Rule: When the string objects are compared by using equals(), then it will compare the contents of the string objects and if we compare the string objects by using == operator, then it will compare the references(hashcodes) of the objects
class Sample {
public static void main(String[] args) {
String s1=1 new String("hello");
String s2= new String("hello");
String s3= hello";
String s4 = "hello";
System.out.println(s1.equals(s2));
System.out.println(s1.equals(s3));
System.out.println(s3.equals(s4));
System.out.println (s1==s2);
System.out.println (s1==s3);
System.out.println (s3==s4);
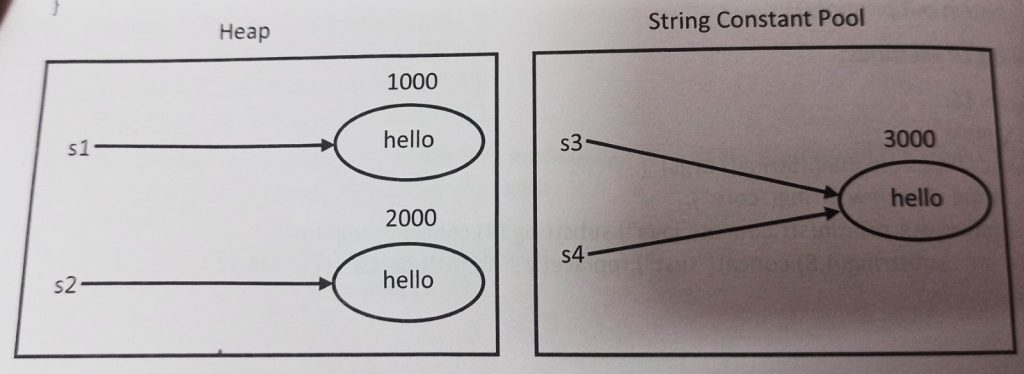