A Service is an app component that can handle long-running operations in the background, and it does not provide a user interface. Another application component can start a service, and it continues to run in the background even if the user switches to another application. Additionally, a component can bind to a service to interact with it and even perform interprocess communication (IPC).
For example, a service can handle network transactions, play music, perform file I/O, or interact with a content provider, all from the background.
These are the three different types of services:
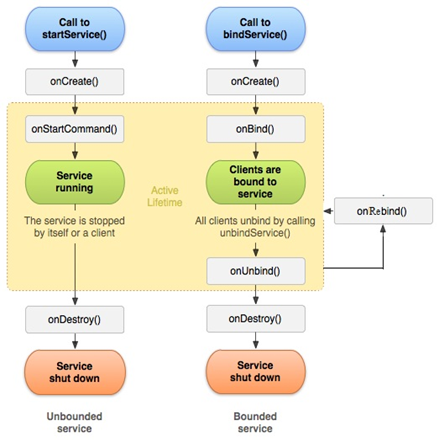
Scheduled
A service is scheduled when an API such as the JobScheduler, introduced in Android 5.0 (API level 21), launches the service. You can use the JobScheduler by registering jobs and specifying their requirements for network and timing. The system then gracefully schedules the jobs for execution at the appropriate times. The JobScheduler provides many methods to define service-execution conditions.
Note: If your app targets Android 5.0 (API level 21), Google recommends that you use the JobScheduler to execute background services. For more information about using this class, see the JobScheduler reference documentation.
Started
A service is started when an application component (such as an activity) calls startService(). After it’s started, a service can run in the background indefinitely, even if the component that started it is destroyed. Usually, a started service performs a single operation and does not return a result to the caller.
For example, it can download or upload a file over the network. When the operation is complete, the service should stop itself.
Bound
A service is bound when an application component binds to it by calling bindService(). A bound service offers a client-server interface that allows components to interact with the service, send requests, receive results, and even do so across processes with interprocess communication (IPC). A bound service runs only as long as another application component is bound to it. Multiple components can bind to the service at once, but when all of them unbind, the service is destroyed.
Regardless of whether your application is started, bound, or both, any application component can use the service (even from a separate application) in the same way that any component can use an activity—by starting it with an Intent. However, you can declare the service as private in the manifest file and block access from other applications. This is discussed more in the section about Declaring the service in the manifest.
Caution: A service runs in the main thread of its hosting process; the service does not create its own thread and does not run in a separate process unless you specify otherwise. If your service is going to perform any CPU-intensive work or blocking operations, such as MP3 playback or networking, you should create a new thread within the service to complete that work. By using a separate thread, you can reduce the risk of Application Not Responding (ANR) errors, and the application’s main thread can remain dedicated to user interaction with your activities.
Activity_mai.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical"
tools:context="androiindians.service.MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello World!" />
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Start Service"
android:id="@+id/bt1"/>
<Button
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Stop Service"
android:id="@+id/bt2"/>
</LinearLayout>
MainActivity.Java
package androiindians.service;
import android.content.Intent;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
public class MainActivity extends AppCompatActivity {
Button button1,button2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
button1= (Button) findViewById(R.id.bt1);
button2= (Button) findViewById(R.id.bt2);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,Myservice.class);
startService(intent);
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent=new Intent(MainActivity.this,Myservice.class);
stopService(intent);
}
});
}
}
Myservice.java
package androiindians.service;
import android.app.Service;
import android.content.Intent;
import android.os.IBinder;
import android.support.annotation.Nullable;
import android.widget.Toast;
public class Myservice extends Service{
@Nullable
@Override
public IBinder onBind(Intent intent) {
return null;
}
@Override
public void onStart(Intent intent, int startId) {
super.onStart(intent, startId);
Toast.makeText(this, "Service was Started", Toast.LENGTH_LONG).show();
}
@Override
public void onCreate() {
super.onCreate();
Toast.makeText(this, "Service was Created", Toast.LENGTH_LONG).show();
}
@Override
public void onDestroy() {
super.onDestroy();
Toast.makeText(this, "Service was Destroyed", Toast.LENGTH_LONG).show();
}
}
Manifestfile.xml
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="androiindians.service">
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
<service android:name=".Myservice"></service>
</application>
</manifest>